Now the real work starts!
So lets continue with our project. First thing what we want is separate some project parts from each other. You want to have some thought about how different parts of program work with each other. So we gonna do so that our WebApp would use reference to other Classes, but not vice-versus. For this, lets create 2 new Class Libaries, lets call them Domain and Services.
Creating Class Libaries
So first, if your project was running then terminate it. Then click on
Solution -> Add -> new Project
From new Project lets create 2 new class Libaries, lets call them ‘Domain’ and ‘Services’. So this is how you find new project creation:
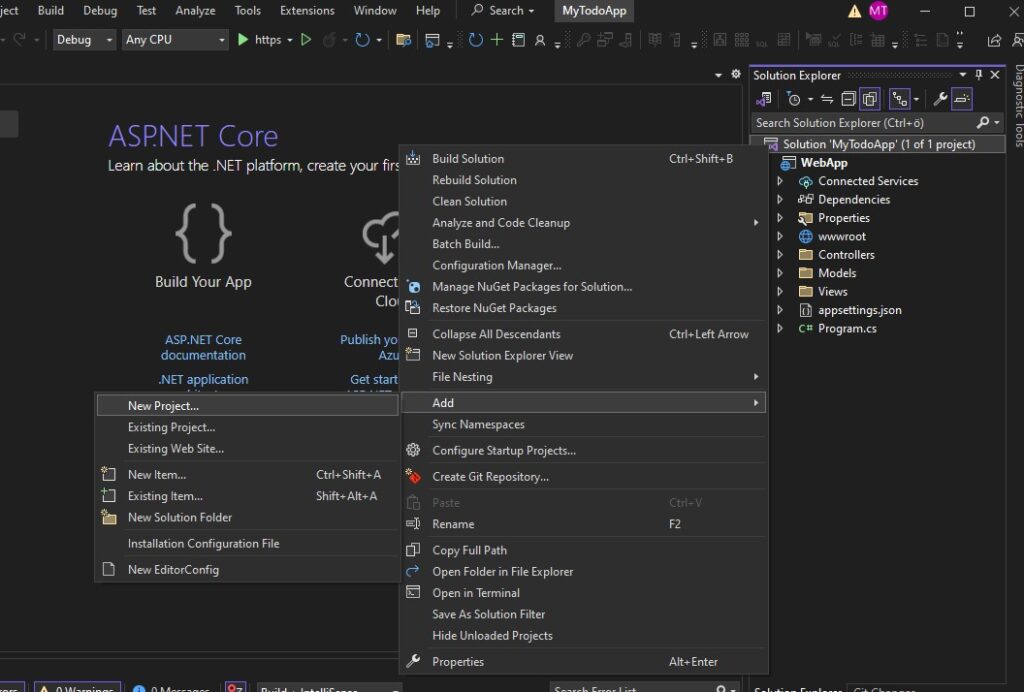
Create 2 new ‘Class Libary’ Type of projects to Solution folder: Domain and Services. If you have completed it your Solution should look like this:
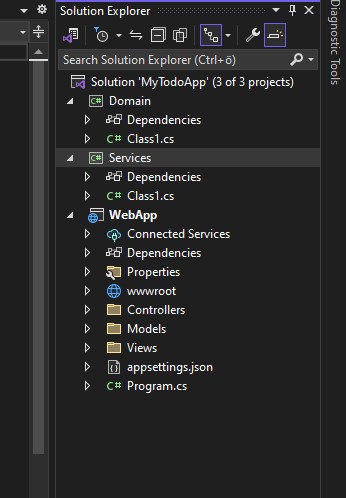
Leave Services project as it is for now, but lets work with Domain project.
Install Necessary Packages
Inside Domain project we would like to include DbContext class and also our model. Toget access to DbContext and Migration functionality, we need to include some necessary Packages
Right Click on Domain ->From there find ‘Manage NuGet Packages’. There will be new View. On that view navigate to Browse folder:
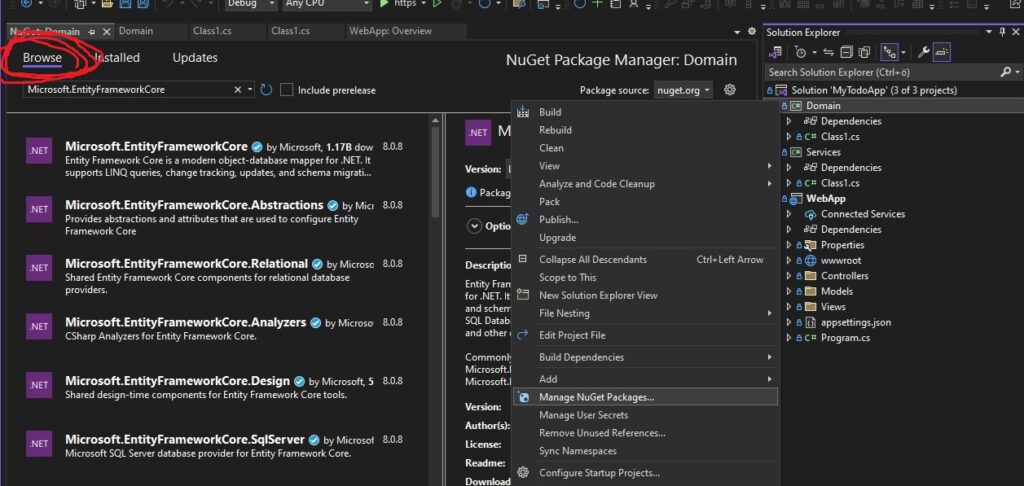
If you managed to find it, you need to install following packages to Domain project:
// Packages for Domain project
Microsoft.EntityFrameworkCore
Microsoft.EntityFrameworkCore.SqlServer
Microsoft.EntityFrameworkCore.Tools
Npgsql.EntityFrameworkCore.PostgreSQL
Since we are already doing package installation, lets include necessary packages for WebApp project as well. Add same Packages to WebApp project as well, and also include CodeGeneration.Design package
// Packages for WebApp project
Microsoft.EntityFrameworkCore
Microsoft.EntityFrameworkCore.SqlServer
Microsoft.EntityFrameworkCore.Tools
Npgsql.EntityFrameworkCore.PostgreSQL
Microsoft.VisualStudio.Web.CodeGeneration.Design
I hope you got it done. Alright, now we can finally start writing some code.
Add Todo class
Inside your Domain folder, remove default Class1.cs and create 3 different files: Todo.cs, Status.cs and AppDbContext.cs. Your domain folder should look like this:
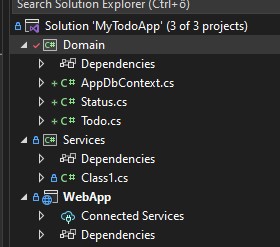
So lets populate these classes. We would like Todo to have a Status: eg InProgress, Pending, Completed. So lets start by creating an enum object to Status.cs file:
// Status.cs:
namespace Domain
{
public enum Status
{
InProgress,
Pending,
Completed
}
}
Now we are ready to populate Todo model with corresponding values. Note that we will be using Attributes as well so after our model is created, then front-end validation is done out of the box. This is our Todo entity:
// Todo.cs
using System.ComponentModel.DataAnnotations;
namespace Domain
{
public class Todo
{
[Key]
public Guid Id { get; set; }
[Required(ErrorMessage = "Title is required")]
[StringLength(100, ErrorMessage = "Title cannot be longer than 100 characters")]
public string? Title { get; set; }
[Required(ErrorMessage = " Description is required")]
[StringLength(500, ErrorMessage = "Description cannot be longer than 500 characters")]
public string? Description { get; set; }
[Required(ErrorMessage = "Due Date is required")]
[DataType(DataType.Date)]
[Display(Name = "Due Date")]
public DateTime DueDate { get; set; }
[Required(ErrorMessage = "Status is required")]
[EnumDataType(typeof(Status), ErrorMessage = "Invalid status value")]
public Status Status { get; set; }
}
}
So for our Todo
we will have Id(type Guid
), Title(string
), Description(string
), DueDate(DateTime
) and Status(our enum
). Now we are ready to write our DbContext class
Writing DbContext class
To AppDbContext class, you need to include using Microsoft.EntityFrameworkCore;
on top of your code. I left it out of this code snippet, but I hope you can include it by yourself. Anyway, main logic for AppDbContext class looks like this:
// AppDbContext.cs
// On top there should be using Microsoft.EntityFrameworkCore; and namespace Domain thing
public class AppDbContext : DbContext
{
public AppDbContext(DbContextOptions<AppDbContext> options) : base(options)
{
}
public DbSet<Todo> Todos { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
base.OnModelCreating(modelBuilder);
}
}
Alright. If you got this done then we are all set to include Migration and new Controller with View. We have completed Model part of MVC part – now lets get database working and include Controller and View part of MVC. Click here to continue building your MVC APP ->
Leave a Reply